AngularJS is an enhanced version of HTML and has become very famous among the web developers. It is pretty straightforward for manipulating the DOM and creating dynamic views in HTML. It is more easier to integrate AngularJS with Bootstarp and build beautiful apps. Bootstarp is another powerful mobile front-end framework for faster and easier web development.
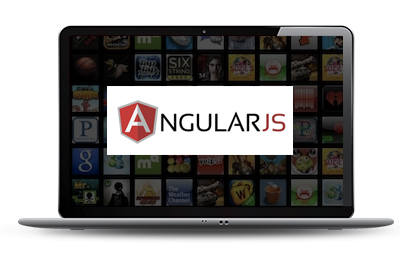
Backend for building Web-Apps with AngularJS – App42 Cloud API
App42 JS APIs can easily be integrated with AngularJS apps for scalable and powerful backend for creating simple to complex web applications. We have created an extended version of AngularJS ToDo example which uses App42 User Management and Storage APIs for login/signup and storing ToDo data on App42 cloud. Here are the quick and easy steps to get started with a great combo of Anguler JS and App42 Backend.
Follow these few steps to get started with App42 JavaScript Backend
- Register with App42 platform.
- Go to the dashboard and click on the Create App button.
- Fill all the mandatory fields and check the ACL = true to get your API_KEY and SECRET_KEY.
- Download source code App42-AngularJS and unzip it on your machine .
- Edit main.js and put your API_KEY and SECRET_KEY here getting from step #3.
- Save and Run index.html file.
Design Details
In this sample I have added two more features, one is login and the other is register to create specific Todos by users using App42JS Framework.
Register Controller
Adding registration feature in this app by fewer lines of code.
function Register ($scope,$injector) {
$scope.word = /^\s*\w*\s*$/;
$scope.setComponent = function(val){
$scope.component = val;
}
$scope.registerUser = function(){
var userName = $scope.registerForm.input.$viewValue,
email = $scope.registerForm.email.$viewValue,
password = $scope.registerForm.password.$viewValue;
showLoading();
new App42User().createUser(userName,password,email,{
success:function(object){
console.log(object)
hideLoading();
$scope.$emit('registerComplete',"registerComplete");
},
error:function(error){
hideLoading();
var errorObj = JSON.parse(error)
var message = errorObj.app42Fault.details;
alert(message);
}
})
};
}
Login Controller
I have added two functions here, one is for authentication and other one is for logout.
function Login ($scope) {
$scope.word = /^\s*\w*\s*$/;
$scope.setComponent = function(val){
$scope.component = val;
$scope.val = true;
}
$scope.$on('registerComplete', function() {
$scope.component = "todo"
$scope.show = {
register: false,
todo: false,
login: false,
logout: true
}
$scope.$apply()
});
/** This function will call when user is successfully logout */
$scope.$on('logoutComplete', function() {
$scope.component = "login"
$scope.show = {
register: true,
todo: false,
login: false,
logout: false
}
$scope.$apply()
});
/** This function will call when user is successfully login */
$scope.$on('loginComplete', function() {
$scope.component = "todo"
$scope.show = {
register: false,
todo: false,
login: false,
logout: true
}
$scope.$apply()
});
$scope.login = function() {
var userName = $scope.myForm.input.$viewValue,
password = $scope.myForm.password.$viewValue;
showLoading();
/** App42 User Login function*/
new App42User().authenticate(userName,password,{
success:function(object){
hideLoading();
$scope.$emit('loginComplete',"loginComplete");
},
error:function(error){
hideLoading();
var errorObj = JSON.parse(error)
var message = errorObj.app42Fault.details;
alert(message);
}
})
};
$scope.logout = function() {
showLoading();
/** App42 User Logout function*/
new App42User().logout(localStorage._App42_SessionId,{
success:function(object){
$scope.$emit('logoutComplete',"logoutComplete");
hideLoading();
},
error:function(error){
hideLoading();
var errorObj = JSON.parse(error)
var message = errorObj.app42Fault.details;
alert(message);
}
})
};
}
Todo Controller
function TodoCrt(){
getTodoList($scope);
$scope.todos = [];
/** This function will call when user is successfully fetch his todos */
$scope.$on('getTodoComplete', function(val,todoObj) {
for (var i=0; i < todoObj.length; i++){
$scope.todos.push(todoObj[i]);
$scope.$apply()
}
})
/** Adding Todos */
$scope.addTodo = function() {
var todotext = $scope.addNew.addTodo.$viewValue
$scope.todos.push({text:todotext, done:false});
var json = JSON.stringify({text:todotext, done:false});
$scope.todoText = '';
addTodoList(json);
};
/** Update Todos */
$scope.updateTodo = function(val,state) {
var newJSON = JSON.stringify({text:val, done:state});
updateTodoList(val,newJSON);
};
$scope.remaining = function() {
var count = 0;
angular.forEach($scope.todos, function(todo) {
count += todo.done ? 0 : 1;
});
return count;
};
}
Saving Your Todo With App42
/** Initialize with App42 */
App42.initialize("API_KEY","SECRET_KEY");
var dbName = "Your Todo DB Name"
var colName = "Your Todo Collection Name"
/** Save your Todos to App42 Cloud by calling this function */
function addTodoList(json){
showLoading();
new App42Storage().insertJSONDocument(dbName,colName,json,{
success:function(object){
var storageObj = JSON.parse(object)
var toList = storageObj.app42.response.storage.jsonDoc
accessDenied(toList._id.$oid)
hideLoading();
},
error:function(error){
hideLoading();
alert("Please check your internet connection.")
}
})
}
/** Fetching Toods From App42 Cloud */
function getTodoList($scope){
showLoading();
new App42Storage().findAllDocuments(dbName,colName,{
success:function(object){
var storageObj = JSON.parse(object)
var toList = storageObj.app42.response.storage.jsonDoc
$scope.$emit('getTodoComplete',toList);
hideLoading();
},
error:function(error){
hideLoading();
alert("You have not created any todo yet.")
}
})
}
/** Update Todo List */
function updateTodoList(val, newJson){
showLoading();
new App42Storage().updateDocumentByKeyValue(dbName,colName,"text",val,newJson,{
success:function(object){
hideLoading();
},
error:function(error){
hideLoading();
alert("Please check your internet connection.")
}
})
}
/** Reemove the access from other users */
function accessDenied(docId){
var aclList = new Array();
var point={
user:"PUBLIC",
permission:Permission.READ
};
aclList.push(point)
new App42Storage().revokeAccessOnDoc(dbName,colName,docId, aclList,{
success: function(object) {
},
error: function(error) {
}
});
}
If you have any questions or need any further assistance to integrate this in your App, please feel free to write us at support@shephertz.com
Leave A Reply